Laravel in Kubernetes Part 1 - Installing Laravel
This series will show you how to go from laravel new
to Laravel running in Kubernetes, including monitoring, logging, exposing and bunch more.
Part 1 of this series covers creating a new laravel installation which we can deploy in Kubernetes.
TLDR;
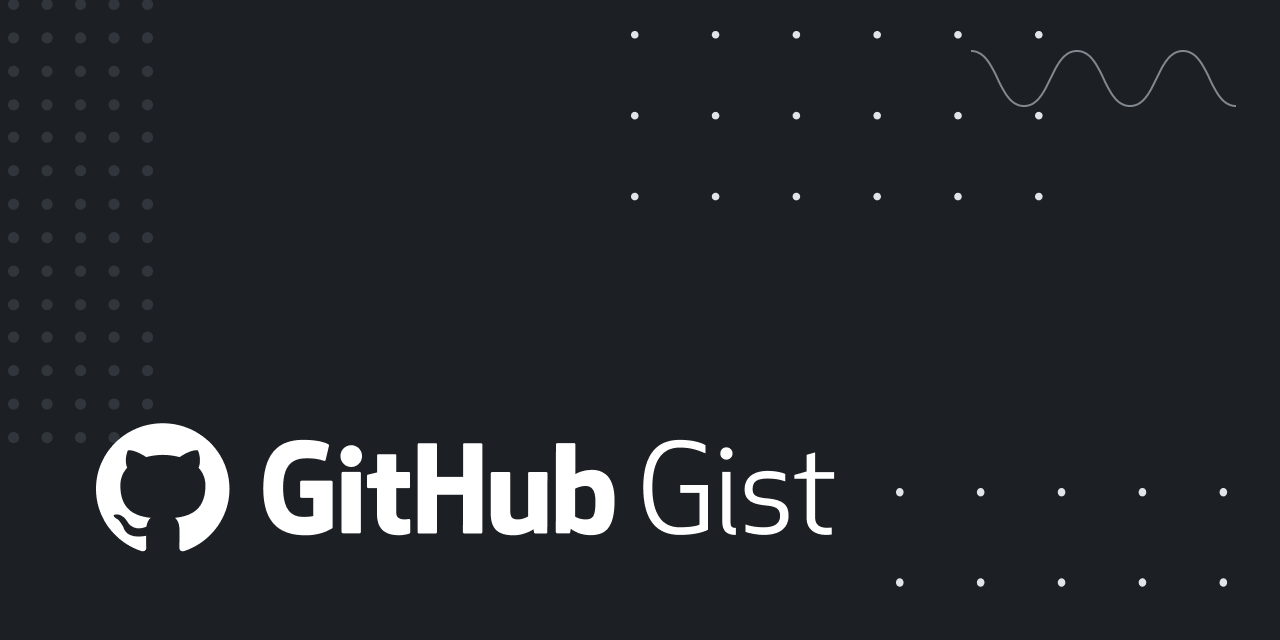
Table of contents
Prerequisites
- Docker running locally.
We will be using Laravel sail to run our application locally as a start, but will build our own Docker images as we go through.
Why ?
- Productionising our Docker images for a smaller size
- We need multiple images for things like fpm and nginx when we move toward running in Kubernetes
- For existing applications which do not have sail as part of their version < 8.0
- Learning
Install a new Laravel application
Change directory to where you want the new application installed.
Install a new Laravel application. For full documentation see here https://laravel.com/docs/8.x/installation#your-first-laravel-project
We will be installing only our app, Redis, and Mysql as part of this post, as we will not be using the rest just yet, and can add them later if necessary.
# Mac OS
curl -s "https://laravel.build/laravel-in-kubernetes?with=mysql,redis" | bash
cd laravel-in-kubernetes
./vendor/bin/sail up
# Linux
curl -s https://laravel.build/laravel-in-kubernetes?with=mysql,redis | bash
cd laravel-in-kubernetes
./vendor/bin/sail up
It might take a while for your application to come up the first time. This is due to new Docker images being downloaded, built, and started up for most services.
You should be able to reach you application http://localhost
Port mappings
Your service might error when starting due to port mounting with an error similar to
ERROR: for laravel.test Cannot start service laravel.test: Ports are not available: listen tcp 0.0.0.0:80: bind: address already in use
To solve this you can set the APP_PORT environment variable when running sail up
APP_PORT=8080 ./vendor/bin/sail up
You should now be able to reach the application at http://localhost:8080 or whichever port you chose in APP_PORT
Understanding the docker-compose file
With sail, your application has a docker-compose.yml
file in the root directory.
This docker-compose file controls what runs when you run sail up
Sail is essentially an abstraction on top of Docker to more easily manage running Laravel
You can see the underlying details by looking at the docker-compose.yml
file, used for running your Laravel application locally, and the ./vendor/laravel/sail/runtimes/8.0/Dockerfile
file, building the container which runs Laravel.
Commit changes
Let's commit our changes at this point, so we can revert anything in future.
git init
git add .
git commit -m "Initial Laravel Install"
Adding authentication
For our application, we want at least a little bit of functionality, so we'll use Laravel Breeze to add a login and register pages.
./vendor/bin/sail composer require laravel/breeze --dev
./vendor/bin/sail php artisan breeze:install
./vendor/bin/sail npm install
./vendor/bin/sail npm run dev
./vendor/bin/sail php artisan migrate
Now you can head over to http://localhost:8080/register to see your new register page.

Fill out the form, submit, and if everything works correctly, you should see a logged in dashboard

Commit again
git add .
git commit -m "Add breeze authentication"
Running tests
You can also run the test suite using
./vendor/bin/sail artisan test
Next, we want to start moving our Laravel application closer to Kubernetes. We will build a bunch of Docker images and update our docker-compose to reflect a more production ready installation.
Onto the next
Next we'll look at Dockerizing our Laravel application for production use