Laravel in Kubernetes Part 3 - Container Registries
In this post, we will take our new Dockerfile and layers, and build the images, and push them up to a registry, so we can easily use them in Kubernetes.
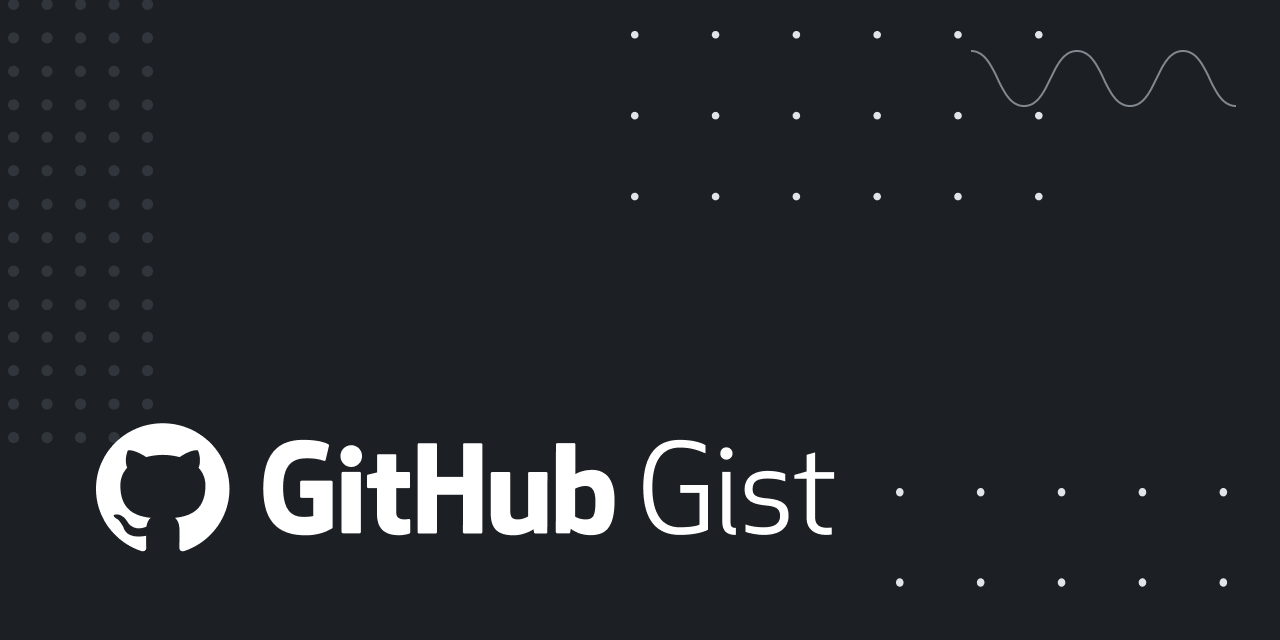
Table of contents
Building our images, and pushing them into a registry
First thing that needs to happen before we can move into Kubernetes, is to build our Docker images containing everything, and ship those to a Container Registry where Kubernetes can reach them.
Docker hub offers free registries, but only 1 private repo.
For our use case we are going to use Gitlab.
It makes it easy to build CI/CD pipelines, as well as has a really nice registry for our images to be stored securely.
Creating the Registry
We need to create a new registry in Gitlab.
If you already have another registry, or prefer using Docker Hub, you may skip this piece.
You'll need a new repository first.
Once you have created one, go to Packages & Registries > Container Registry, and you'll see instructions on how to login, and get the url for your container registry
In my case this is registry.gitlab.com/laravel-in-kubernetes/laravel-app
Login to the registry
Depending on whether you have 2 factor auth enabled, you might need to generate credentials for your local machine.
You can create a pair in Settings > Repository > Deploy Tokens, and use these as a username and password to login to the registry. The Deploy Token needs write access to the registry.
$ docker login registry.gitlab.com -u [username] -p [token]
Login Succeeded
Building our images
We now need to build our application images, and tag them to our registry.
In order to do this we need to point at the specific stage we need to build, and tag it with a name.
$ docker build . -t [your_registry_url]/cli:v0.0.1 --target cli
$ docker build . -t [your_registry_url]/fpm_server:v0.0.1 --target fpm_server
$ docker build . -t [your_registry_url]/web_server:v0.0.1 --target web_server
$ docker build . -t [your_registry_url]/cron:v0.0.1 --target cron
Pushing our images
Next we need to push our images to our new registry to be used with Kubernetes
$ docker push [your_registry_url]/cli:v0.0.1
$ docker push [your_registry_url]/fpm_server:v0.0.1
$ docker push [your_registry_url]/web_server:v0.0.1
$ docker push [your_registry_url]/cron:v0.0.1
Our images are now available inside the registry, and ready to be used in Kubernetes.
Repeatable build steps with Makefile
In order for us to easily repeat the build steps, we can use a Makefile to specify our build commands, and variableise the specific pieces like our registry url, and the version of our containers.
In the root of the project, create a Makefile
$ touch Makefile
This file will allow us to express our build commands reproducibly.
In the new Makefile
add the following contents, which variableise the version and registry, and then specify the commands.
# VERSION defines the version for the docker containers.
# To build a specific set of containers with a version,
# you can use the VERSION as an arg of the docker build command (e.g make docker VERSION=0.0.2)
VERSION ?= v0.0.1
# REGISTRY defines the registry where we store our images.
# To push to a specific registry,
# you can use the REGISTRY as an arg of the docker build command (e.g make docker REGISTRY=my_registry.com/username)
# You may also change the default value if you are using a different registry as a default
REGISTRY ?= registry.gitlab.com/laravel-in-kubernetes/laravel-app
# Commands
docker: docker-build docker-push
docker-build:
docker build . --target cli -t ${REGISTRY}/cli:${VERSION}
docker build . --target cron -t ${REGISTRY}/cron:${VERSION}
docker build . --target fpm_server -t ${REGISTRY}/fpm_server:${VERSION}
docker build . --target web_server -t ${REGISTRY}/web_server:${VERSION}
docker-push:
docker push ${REGISTRY}/cli:${VERSION}
docker push ${REGISTRY}/cron:${VERSION}
docker push ${REGISTRY}/fpm_server:${VERSION}
docker push ${REGISTRY}/web_server:${VERSION}
You can then use a make command to easily build, and push the containers all together.
$ make docker VERSION=v0.0.2
# If you only want to run the builds
$ make docker-build VERSION=v0.0.2
# If you only want to push the images
$ make docker-push VERSION=v0.0.2
Onto the next
Next we will setup our Kubernetes Cluster where we will run our images.